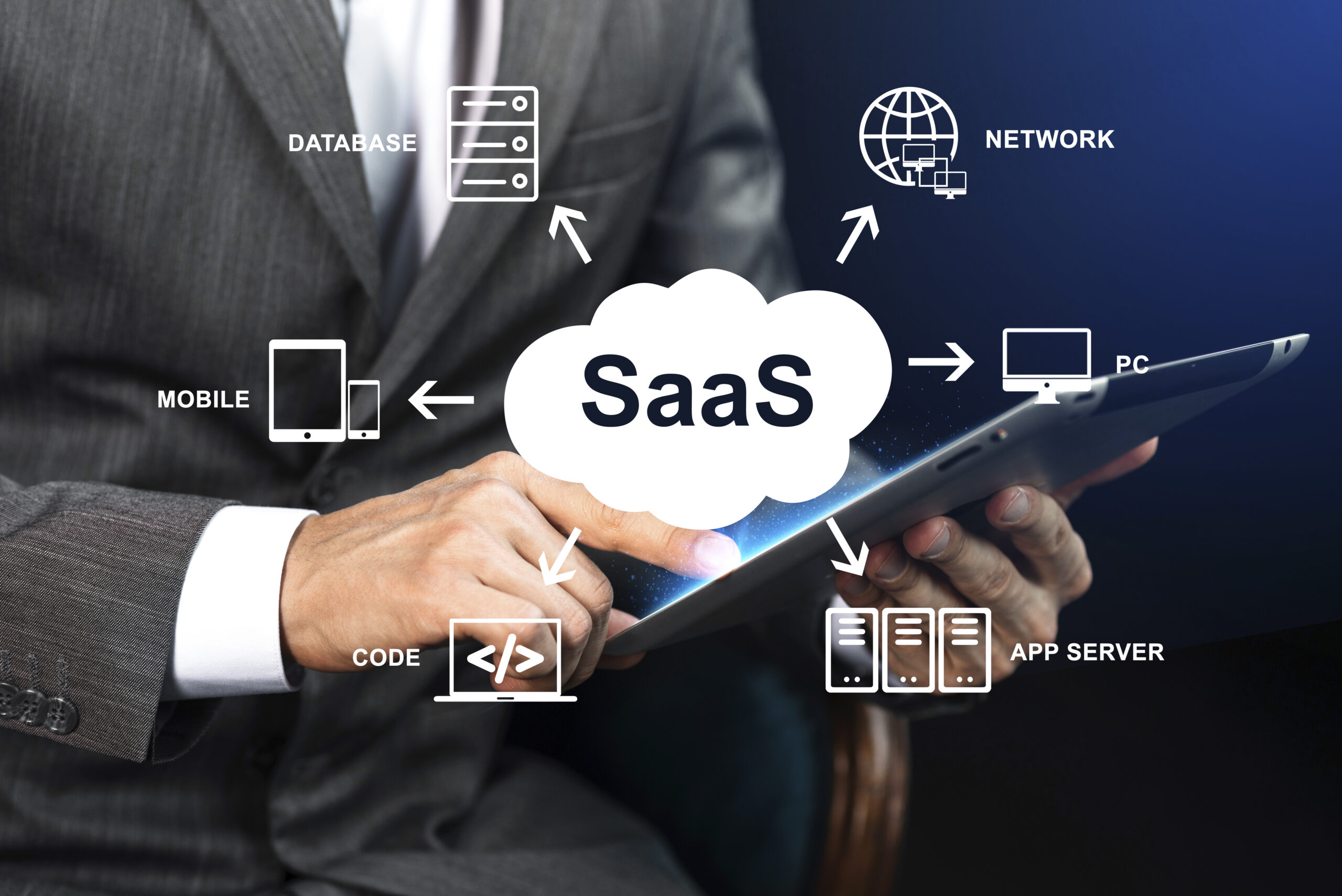
Creating a scalable Software as a Service (SaaS) application with Laravel is a winning move for developers aiming for efficiency and growth. Laravel’s powerful features and clean design make it ideal for SaaS projects. This guide cuts through the noise to deliver clear, actionable steps.
Let’s jump right in.
Step 1: Plan Your SaaS Foundation
Start by mapping out your SaaS essentials:
- Multi-Tenancy: Decide between one database with tenant separation or unique databases per tenant. One database is easier to manage; separate ones boost isolation.
- Core Features: Think subscriptions, user logins, payment systems, and dashboards.
- Growth Strategy: Design for scalability—optimize queries and prepare for extra servers.
Quick Tip: Leverage Laravel’s Eloquent ORM and migrations for a solid base.
Step 2: Launch Your Laravel Project
Kick off with a clean Laravel setup:
Install via Composer:
Tweak your .env file with database details and app settings.
Initialize a Git repository to monitor your progress:
SEO Boost: A smooth start paves the way for rapid scaling—readers love that in a Laravel SaaS tutorial.
Step 3: Set Up Multi-Tenancy
Multi-tenancy is key for SaaS. Here’s how Laravel handles it:
- Single Database:
- Add a tenant_id to tables.
Scope queries with middleware:
Separate Databases:
- Dynamically switch connections:
php
Step 4: Add Authentication and Access Control
Secure your SaaS with Laravel’s tools:
- Use Laravel Breeze or Jetstream for instant auth scaffolding:
- Tie registration to tenants or subscription tiers.
- Control permissions with Gates
Why It’s Crucial: Strong security builds user trust fast.
Step 5: Enable Subscriptions with Cashier
Add recurring payments easily:
Install Laravel Cashier:
- Define plans in config/services.php.
- Implement subscriptions:
Growth Hack: Use webhooks to process payments in the background, keeping your app snappy.
Step 6: Boost Scalability
Prepare your SaaS for heavy traffic:
- Caching: Store frequent data with Redis or Memcached.
- Queues: Offload tasks like notifications:
- Load Balancing: Sync sessions with Redis for multi-server setups.
Step 7: Test Thoroughly
Ensure reliability before launch:
- Write tests with PHPUnit:
- Test UIs with Laravel Dusk if needed.
SEO Win: Tested apps rank higher with readers searching “Laravel SaaS development guide.”
Step 8: Deploy and Track Performance
- Launch on AWS, DigitalOcean, or Laravel Forge.
- Automate deployments with GitHub Actions or Envoyer.
- Monitor with Laravel Telescope or external tools like New Relic.
Pro Move: Log errors with Laravel’s system to stay ahead of issues.
Wrap-Up
With Laravel, building a scalable SaaS is fast and effective. Plan smart, use its built-in tools, and optimize for growth. Whether you’re aiming small or big, Laravel scales with you.
Start coding now—your SaaS awaits!