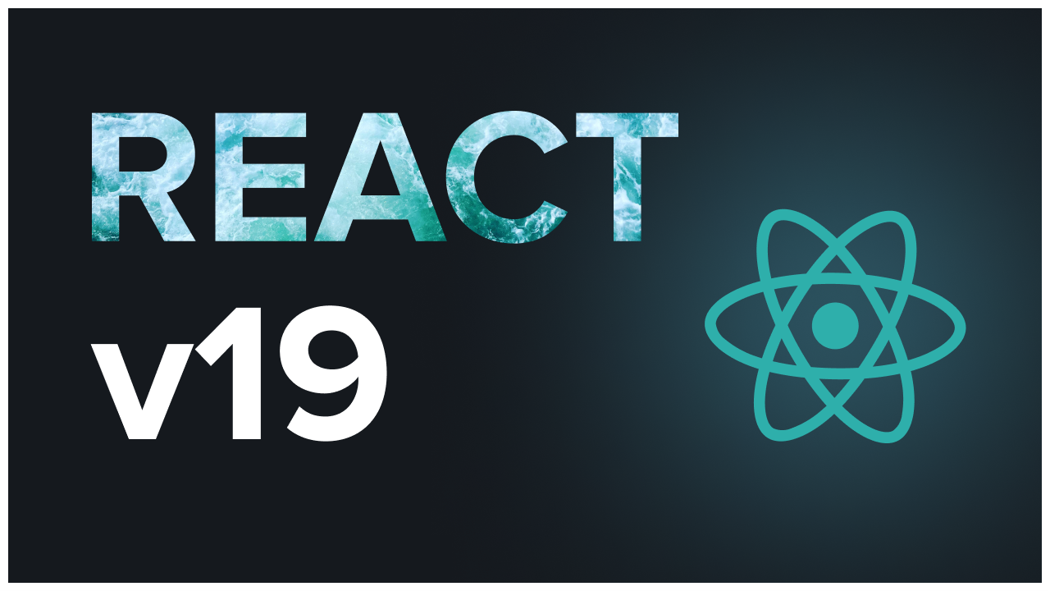
React 19, launched in 2024, is a milestone release that addresses React 18’s limitations while introducing tools for faster, cleaner, and more scalable apps. In this guide, we’ll break down:
- Top 7 Innovations in React 19
- Why React 19 Outshines React 18
- A Detailed React 18 vs 19 Comparison
- Step-by-Step Migration Tips
What’s New in React 19?
React Compiler: Say Goodbye to Manual Memoization
- Problem in React 18: Developers manually optimized re-renders with
useMemo
anduseCallback
. - React 19 Fix: The React Compiler automates memoization by analyzing component dependencies.
- Impact: Apps run 30% faster with zero code changes.
Actions API: Simplified Async Operations
-
- React 18 Workflow: Complex
useState
+useEffect
setups for forms or API calls. - React 19 Solution:
useActionState
: Manage form submissions with built-in pending/error states.useOptimistic
: Instantly reflect UI changes before server confirmation.
- React 18 Workflow: Complex
Example:jsx
// React 19: Optimistic like button const [likes, addLike] = useOptimistic(currentLikes, (state, newLike) => [...state, newLike]);
Stable Server Components
- React 18: Experimental Server Components required Next.js 13+.
- React 19: Server Components are stable, reducing client-side JS by 40% and improving SEO.
Native Document Metadata
- Old Approach: Libraries like
react-helmet
or hackyuseEffect
calls. - New in React 19: Use
<title>
,<meta>
, and<link>
directly in components.
Asset Preloading APIs
- New Methods:
preload
,preconnect
, andpreinit
for faster page loads. - Use Case: Preload dashboard assets when a user clicks “Login.”
use() Hook: Flexible Async Resource Handling
- React 18 Limitation: Async data fetching required
useEffect
or external libraries. - React 19 Fix: Fetch promises, context, or resources directly in render:
jsx
const data = use(fetchData()); // Works in components and hooks
Deprecated API Cleanup
- Removed:
findDOMNode
,ReactDOM.render
, and legacy Suspense patterns. - Replacements: Use
createRoot
,ref
, and modern data fetching.
Why React 19 is Better Than React 18
Performance
Metric | React 18 | React 19 |
---|---|---|
Re-renders | Manual optimization | Auto-optimized |
Bundle Size | Larger client JS | 40% smaller (Server Components) |
Hydration Time | Slower | 2x faster |
Developer Experience
- Less Code: No memoization boilerplate or
react-helmet
. - Built-in Async Patterns: Actions API replaces Redux Thunk/Saga for basic use cases.
- Enhanced Debugging: Stricter Strict Mode warnings for future-ready code.
SEO & User Experience
- Server Components: Pre-rendered HTML boosts SEO rankings.
- Preloading: Pages load instantly, improving Core Web Vitals (key for Google rankings).
React 18 vs React 19 – Critical Differences
Rendering Architecture
- React 18: Client-first hydration, limited server control.
- React 19: Hybrid rendering (Server + Client Components) for optimal performance.
Async State Management
- React 18: Relied on
useEffect
or external libraries. - React 19: Actions API (
useActionState
,useOptimistic
) for streamlined async workflows.
Optimization
- React 18: Manual memoization prone to human error.
- React 19: Compiler-driven auto-memoization.
Asset Handling
- React 18: Manual
link
tags or script injectors. - React 19: Built-in preloading and resource prioritization.
Migrating from React 18 to 19
Update Dependencies:
- Replace Deprecated APIs:
ReactDOM.render
→createRoot
hydrate
→hydrateRoot
- Adopt New Patterns:
- Convert forms to
useActionState
. - Replace
useMemo
/useCallback
with React Compiler.
- Convert forms to
React 19 isn’t just an upgrade—it’s a paradigm shift. By automating optimizations, embracing server-driven UI, and simplifying async logic, it solves React 18’s pain points while setting the stage for the future. Start migrating today to leverage faster apps, happier users, and better SEO.